A Technical Walkthrough: Deploying and Managing Containers with AWS ECS and Fargate
A Technical Walkthrough: Deploying and Managing Containers with AWS ECS and Fargate Containers are the backbone of modern cloud-native applications, offering portability, scalability, and efficiency. AWS provides multiple container orchestration tools, but in this walkthrough, we’ll focus on Amazon Elastic Container Service (ECS) with AWS Fargate, showcasing how to deploy and manage a containerized application step-by-step. Prerequisites Before diving into the walkthrough, ensure you have the following: An AWS account with admin permissions. The AWS CLI installed and configured. Docker installed for building container images. Basic knowledge of containers and Docker. Step 1: Build and Push Your Docker Image to Amazon ECR 1.1: Create a Dockerfile Let’s create a simple Dockerfile for a Python web application using Flask: # Use an official Python runtime as the base image FROM python:3.9-slim # Set the working directory WORKDIR /app # Copy application files COPY requirements.txt requirements.txt COPY app.py app.py # Install dependencies RUN pip install -r requirements.txt # Expose the application port EXPOSE 5000 # Define the command to run the application CMD ["python", "app.py"] 1.2: Build and Tag the Image Run the following commands in your terminal: docker build -t flask-app . 1.3: Push the Image to Amazon ECR Create a Repository: aws ecr create-repository --repository-name flask-app Authenticate Docker to ECR: aws ecr get-login-password --region | docker login --username AWS --password-stdin .dkr.ecr..amazonaws.com Tag the Image: docker tag flask-app:latest .dkr.ecr..amazonaws.com/flask-app:latest Push the Image: docker push .dkr.ecr..amazonaws.com/flask-app:latest Step 2: Create an ECS Cluster Create the Cluster: aws ecs create-cluster --cluster-name flask-cluster Verify the Cluster: aws ecs list-clusters Step 3: Define a Task Definition A task definition specifies how containers should run in ECS. Create a Task Definition JSON File: Save the following as task-definition.json: { "family": "flask-task", "networkMode": "awsvpc", "executionRoleArn": "arn:aws:iam:::role/ecsTaskExecutionRole", "containerDefinitions": [ { "name": "flask-container", "image": ".dkr.ecr..amazonaws.com/flask-app:latest", "memory": 512, "cpu": 256, "essential": true, "portMappings": [ { "containerPort": 5000, "hostPort": 5000 } ] } ], "requiresCompatibilities": ["FARGATE"], "cpu": "256", "memory": "512" } Register the Task Definition: aws ecs register-task-definition --cli-input-json file://task-definition.json Step 4: Run a Service with Fargate Create a Service: aws ecs create-service \ --cluster flask-cluster \ --service-name flask-service \ --task-definition flask-task \ --desired-count 1 \ --launch-type FARGATE \ --network-configuration "awsvpcConfiguration={subnets=[],securityGroups=[],assignPublicIp=ENABLED}" Verify the Service: aws ecs list-services --cluster flask-cluster Step 5: Test the Application Retrieve the public IP of your running task: List Tasks: aws ecs list-tasks --cluster flask-cluster Describe the Task: aws ecs describe-tasks --cluster flask-cluster --tasks Access the Application: Open the public IP in your browser on port 5000. You should see your Flask app running. Step 6: Monitor and Scale Enable CloudWatch Logs: Add the following to your task-definition.json under containerDefinitions: "logConfiguration": { "logDriver": "awslogs", "options": { "awslogs-group": "/ecs/flask-service", "awslogs-region": "", "awslogs-stream-prefix": "ecs" } } Update the task definition and re-deploy the service to enable logging. Scale the Service: aws ecs update-service --cluster flask-cluster --service flask-service --desired-count 3 Conclusion In this walkthrough, we’ve deployed a containerized Flask application to AWS ECS using Fargate. By leveraging AWS’s fully managed services, we’ve minimized the operational overhead and focused entirely on the application. As you grow, explore features like auto-scaling, service discovery, and integration with CI/CD pipelines to optimize your deployments further. Feel free to share your thoughts, or let me know if you encounter any challenges while implementing this workflow!

A Technical Walkthrough: Deploying and Managing Containers with AWS ECS and Fargate
Containers are the backbone of modern cloud-native applications, offering portability, scalability, and efficiency. AWS provides multiple container orchestration tools, but in this walkthrough, we’ll focus on Amazon Elastic Container Service (ECS) with AWS Fargate, showcasing how to deploy and manage a containerized application step-by-step.
Prerequisites
Before diving into the walkthrough, ensure you have the following:
- An AWS account with admin permissions.
- The AWS CLI installed and configured.
- Docker installed for building container images.
- Basic knowledge of containers and Docker.
Step 1: Build and Push Your Docker Image to Amazon ECR
1.1: Create a Dockerfile
Let’s create a simple Dockerfile for a Python web application using Flask:
# Use an official Python runtime as the base image
FROM python:3.9-slim
# Set the working directory
WORKDIR /app
# Copy application files
COPY requirements.txt requirements.txt
COPY app.py app.py
# Install dependencies
RUN pip install -r requirements.txt
# Expose the application port
EXPOSE 5000
# Define the command to run the application
CMD ["python", "app.py"]
1.2: Build and Tag the Image
Run the following commands in your terminal:
docker build -t flask-app .
1.3: Push the Image to Amazon ECR
- Create a Repository:
aws ecr create-repository --repository-name flask-app
- Authenticate Docker to ECR:
aws ecr get-login-password --region | docker login --username AWS --password-stdin .dkr.ecr..amazonaws.com
- Tag the Image:
docker tag flask-app:latest .dkr.ecr..amazonaws.com/flask-app:latest
- Push the Image:
docker push .dkr.ecr..amazonaws.com/flask-app:latest
Step 2: Create an ECS Cluster
- Create the Cluster:
aws ecs create-cluster --cluster-name flask-cluster
- Verify the Cluster:
aws ecs list-clusters
Step 3: Define a Task Definition
A task definition specifies how containers should run in ECS.
-
Create a Task Definition JSON File: Save the following as
task-definition.json
:
{
"family": "flask-task",
"networkMode": "awsvpc",
"executionRoleArn": "arn:aws:iam:::role/ecsTaskExecutionRole" ,
"containerDefinitions": [
{
"name": "flask-container",
"image": ".dkr.ecr..amazonaws.com/flask-app:latest" ,
"memory": 512,
"cpu": 256,
"essential": true,
"portMappings": [
{
"containerPort": 5000,
"hostPort": 5000
}
]
}
],
"requiresCompatibilities": ["FARGATE"],
"cpu": "256",
"memory": "512"
}
- Register the Task Definition:
aws ecs register-task-definition --cli-input-json file://task-definition.json
Step 4: Run a Service with Fargate
- Create a Service:
aws ecs create-service \
--cluster flask-cluster \
--service-name flask-service \
--task-definition flask-task \
--desired-count 1 \
--launch-type FARGATE \
--network-configuration "awsvpcConfiguration={subnets=[],securityGroups=[],assignPublicIp=ENABLED}"
- Verify the Service:
aws ecs list-services --cluster flask-cluster
Step 5: Test the Application
Retrieve the public IP of your running task:
- List Tasks:
aws ecs list-tasks --cluster flask-cluster
- Describe the Task:
aws ecs describe-tasks --cluster flask-cluster --tasks
-
Access the Application:
Open the public IP in your browser on port
5000
. You should see your Flask app running.
Step 6: Monitor and Scale
-
Enable CloudWatch Logs:
Add the following to your
task-definition.json
undercontainerDefinitions
:
"logConfiguration": {
"logDriver": "awslogs",
"options": {
"awslogs-group": "/ecs/flask-service",
"awslogs-region": "" ,
"awslogs-stream-prefix": "ecs"
}
}
Update the task definition and re-deploy the service to enable logging.
- Scale the Service:
aws ecs update-service --cluster flask-cluster --service flask-service --desired-count 3
Conclusion
In this walkthrough, we’ve deployed a containerized Flask application to AWS ECS using Fargate. By leveraging AWS’s fully managed services, we’ve minimized the operational overhead and focused entirely on the application. As you grow, explore features like auto-scaling, service discovery, and integration with CI/CD pipelines to optimize your deployments further.
Feel free to share your thoughts, or let me know if you encounter any challenges while implementing this workflow!
What's Your Reaction?
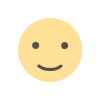
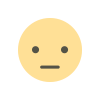
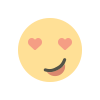
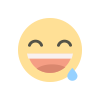
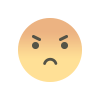
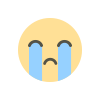
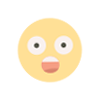